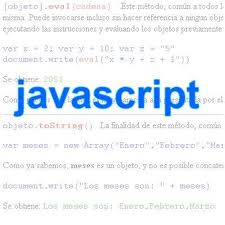
So what's a loop then? A loop is something that goes round and round. If I told you to move a finger around in a loop, you'd have no problem with the order (unless you have no fingers!) In programming, it's exactly the same. Except a programming loop will go round and round until you tell it to stop. You also need to tell the programme two other things - where to start your loop, and what to do after it's finished one lap (known as the update expression).
There are three types of loops in JavaScript: for loops, while loops, and do … while loops. We'll start with the most common type - the for loop.
For Loops
Here's a JavaScript for loop in a little script. Type, or copy and paste, it into the HEAD section of web page (along with the script tags) and test it out.
counter = 0
for(start = 1; start < 10; start++) {
counter = counter + 1
document.write("start = " + start + " counter = " + counter + "<BR>")
}
How did you get on? You should have this printed on your page:
start = 1 counter = 1
start = 2 counter = 2
start = 3 counter = 3
start = 4 counter = 4
start = 5 counter = 5
start = 6 counter = 6
start = 7 counter = 7
start = 8 counter = 8
start = 9 counter = 9
start = 10 counter = 10
The format for a for loop is this:
for (start value; end value; update expression) {
}
The first thing you need to do is type the name of the loop you're using, in this case for (in lower case letters). In between round brackets, you then type your three conditions:
Start Value
The first condition is where you tell JavaScript the initial value of your loop. In other words, start the loop at what number? We used this:
start = 1
We're assigning a value of 1 to a variable called start. Like all variables, you can make up your own name. A popular name for the initial variable is the letter i . You can set the initial condition before the loop begins. Like this:
start = 1
for(start; start < 11; start++) {
The result is the same - the start number for the loop is 1
End Value
Next, you have to tell JavaScript when to end your loop. This can be a number, a Boolean value, a string, etc. Here, we're telling JavaScript to bail out of the loop when the value of the variable start is Less Than 11.
Update Expression
Loops need a way of getting the next number in a series. If the loop couldn't update the starting value, it would be stuck on the starting value. If we didn't update our start value, our loop would get stuck on 1. In other words, you need to tell the loop how it is to go round and round. We used this:
start++
In the java programming language the double plus symbol (++) means increment (increase the value by one). It's just a short way of saying this:
start = start + 1
You can go down by one (decrement) by using the double minus symbol (--), but we won't go into that.
So our whole loop reads "Starting at a value of 1, keep going round and round until the start value is less than 11. Increase the starting value by one each time round the loop."
Every time the loop goes round, the code between our two curly brackets { } gets executed:
counter = counter + 1
document.write("start = " + start + " counter = " + counter + "<BR>")
Notice that we're just incrementing the counter variable by 1 each time round the loop, exactly the same as what we're doing with the start variable. So we could have put this instead:
counter++
The effect would be the same. As an experiment, try setting the value of the counter to 11 outside the loop (it's currently counter = 0). Then inside the loop, use counter- - (the double minus sign).